
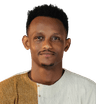
Denamo Markos
Smart Contract — Employee Tracking
Overview
The employee tracking smart contract is aimed to be used when one party, for example, an employer agrees to pay another party, for example, an employee, for being present in a certain geographic area for a certain duration. The employee's phone sends its GPS location to a smart contract at a certain interval. Based on the pre-agreed contract codified in an Ethereum smart contract, a cryptocurrency payment is executed when all the agreed conditions are met.
Info: If at any point, the GPS sensor indicates that an employee is outside the range of the agreed GPS area, the contract state will be updated to indicate that it is out of compliance.
Technologies and Tools
Core Technologies
Technology | Purpose | Description |
---|---|---|
Solidity | Smart Contract | Programming language for Ethereum smart contracts |
Remix IDE | Development | IDE for smart contract development and deployment |
Hardhat | Framework | Ethereum development environment |
Truffle | Framework | Development environment and testing framework |
Infura | Infrastructure | Tools for blockchain application deployment |
React | Frontend | UI library for web application |
Flutter | Mobile | Cross-platform mobile development SDK |
Implementation
Contract
The solidity smart contract has several parameters, a constructor, and methods related to location and employee.
1// Structs and contract variables 2struct Employee { 3 address employeeAddress; 4 string name; 5 bool isActive; 6} 7 8struct Location { 9 int256 latitude; 10 int256 longitude; 11 uint256 timestamp; 12} 13 14address public owner; 15mapping(address => Employee) public employees; 16Location public officeLocation; 17uint256 public radius; 18 19// Contract constructor 20constructor() { 21 owner = msg.sender; 22 radius = 100; // Default radius in meters 23} 24 25// Location methods 26function setLocation(int256 _lat, int256 _long) public onlyOwner { 27 officeLocation.latitude = _lat; 28 officeLocation.longitude = _long; 29 officeLocation.timestamp = block.timestamp; 30} 31 32function setRadius(uint256 _radius) public onlyOwner { 33 radius = _radius; 34}
Web App (Admin)
Here the admins are able to:
- Add new employees
- Get employee information
- Set office location and radius
- Set payable amount
Mobile App (Employee)
The mobile application is used to report employee location:
- Reads user's location
- Reports location to smart contract
- Location checked for range compliance
- Processes payment if conditions met
Lesson Learned
By taking on this challenge I was able to learn new concepts:
- Understanding of Ethereum blockchain and smart contracts
- Experience with Ethereum development environments (hardhat, truffle)
- Building React web app and Flutter mobile app integration with smart contracts
Info: The project provided hands-on experience with blockchain development and cross-platform application integration.
Future Plan
Future works that could help grow this project:
- Smart Contract Improvements
- Enhanced implementation
- Additional methods
- Better security features
- Application Enhancements
- Extended employee management features
- Improved user experience
- Additional mobile app features
- Deployment
- Testnet deployment
- Production environment setup
- Performance optimization